プログラミングできても画面デザインは頑張れません。
そういう人にマテリアルデザインはおススメです。
WPF でマテリアルデザインをするための土台作りの手順
https://qiita.com/okazuki/items/7596ec596cad00900faf
※サンプルそのままで行けなかった部分があったので、記事にしました。
Nugetパッケージ
以下を導入します。
- MaterialDesignThemes.MahApps
- MahApps.Metro
App.xaml
以下は黒背景の例です。
<Application x:Class="Sample.App"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:Sample"
StartupUri="MainWindow.xaml">
<Application.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<ResourceDictionary Source="pack://application:,,,/MahApps.Metro;component/Styles/Controls.xaml" />
<!-- Material Design -->
<ResourceDictionary Source="pack://application:,,,/MaterialDesignColors;component/Themes/Recommended/Primary/MaterialDesignColor.Amber.xaml"/>
<ResourceDictionary Source="pack://application:,,,/MaterialDesignColors;component/Themes/Recommended/Secondary/MaterialDesignColor.Cyan.xaml" />
<ResourceDictionary Source="pack://application:,,,/MaterialDesignThemes.Wpf;component/Themes/MaterialDesignTheme.Dark.xaml" />
<ResourceDictionary Source="pack://application:,,,/MaterialDesignThemes.Wpf;component/Themes/MaterialDesign3.Defaults.xaml" />
<!-- MahApps -->
<ResourceDictionary Source="pack://application:,,,/MahApps.Metro;component/Styles/Fonts.xaml" />
<ResourceDictionary Source="pack://application:,,,/MahApps.Metro;component/Styles/Themes/Dark.Violet.xaml" />
<!-- Material Design: MahApps Compatibility -->
<ResourceDictionary Source="pack://application:,,,/MaterialDesignThemes.MahApps;component/Themes/MaterialDesignTheme.MahApps.Fonts.xaml" />
<ResourceDictionary Source="pack://application:,,,/MaterialDesignThemes.MahApps;component/Themes/MaterialDesignTheme.MahApps.Flyout.xaml" />
</ResourceDictionary.MergedDictionaries>
<!-- MahApps Brushes -->
<SolidColorBrush x:Key="MahApps.Brushes.Highlight" Color="{DynamicResource Primary700}" />
<SolidColorBrush x:Key="MahApps.Brushes.AccentBase" Color="{DynamicResource Primary600}" />
<SolidColorBrush x:Key="MahApps.Brushes.Accent" Color="{DynamicResource Primary500}" />
<SolidColorBrush x:Key="MahApps.Brushes.Accent2" Color="{DynamicResource Primary400}" />
<SolidColorBrush x:Key="MahApps.Brushes.Accent3" Color="{DynamicResource Primary300}" />
<SolidColorBrush x:Key="MahApps.Brushes.Accent4" Color="{DynamicResource Primary200}" />
<SolidColorBrush x:Key="MahApps.Brushes.WindowTitle" Color="{DynamicResource Primary700}" />
<SolidColorBrush x:Key="MahApps.Brushes.Selected.Foreground" Color="{DynamicResource Primary500Foreground}" />
<LinearGradientBrush x:Key="MahApps.Brushes.Progress" StartPoint="1.002,0.5" EndPoint="0.001,0.5">
<GradientStop Offset="0" Color="{DynamicResource Primary700}" />
<GradientStop Offset="1" Color="{DynamicResource Primary300}" />
</LinearGradientBrush>
<SolidColorBrush x:Key="MahApps.Brushes.CheckmarkFill" Color="{DynamicResource Primary500}" />
<SolidColorBrush x:Key="MahApps.Brushes.RightArrowFill" Color="{DynamicResource Primary500}" />
<SolidColorBrush x:Key="MahApps.Brushes.IdealForeground" Color="{DynamicResource Primary500Foreground}" />
<SolidColorBrush
x:Key="MahApps.Brushes.IdealForegroundDisabled"
Opacity="0.4"
Color="{DynamicResource Primary500}" />
<!--#region MaterialDesign ScrollBar-->
<Style x:Key="MaterialDesignScrollBarButtonStyle" TargetType="RepeatButton" BasedOn="{StaticResource MaterialDesignScrollBarButton}"/>
<Style x:Key="MaterialDesignRepeatButtonTransparentStyle" TargetType="RepeatButton" BasedOn="{StaticResource MaterialDesignRepeatButtonTransparent}"/>
<Style x:Key="MaterialDesignScrollBarThumbStyle" TargetType="Thumb" BasedOn="{StaticResource MaterialDesignScrollBarThumb}"/>
<!--<Style x:Key="MaterialDesignScrollBarThumbVerticalStyle" TargetType="Thumb" BasedOn="{StaticResource MaterialDesignScrollBarThumbVertical}"/>
<Style x:Key="MaterialDesignScrollBarThumbHorizontalStyle" TargetType="Thumb" BasedOn="{StaticResource MaterialDesignScrollBarThumbHorizontal}"/>-->
<Style x:Key="MaterialDesignScrollBarStyle" TargetType="ScrollBar" BasedOn="{StaticResource MaterialDesignScrollBar}"/>
<Style TargetType="ScrollBar" BasedOn="{StaticResource MaterialDesignScrollBarMinimal}"/>
<!--#endregion-->
<!--#region MaterialDesign ScrollViewer-->
<Style x:Key="MaterialDesignScrollViewerStyle" TargetType="ScrollViewer" BasedOn="{StaticResource MaterialDesignScrollViewer}"/>
<Style TargetType="TextBlock" BasedOn="{StaticResource MahApps.Styles.TextBlock}">
<Style.Setters>
<Setter Property="Foreground" Value="White"/>
</Style.Setters>
</Style>
<Style TargetType="TextBox" BasedOn="{StaticResource MaterialDesignTextBox}">
<Style.Setters>
<Setter Property="Foreground" Value="White"/>
</Style.Setters>
</Style>
<Style TargetType="ToolTip" BasedOn="{StaticResource MahApps.Styles.ToolTip}">
<Style.Setters>
<Setter Property="Foreground" Value="Black"/>
</Style.Setters>
</Style>
<!--#endregion-->
</ResourceDictionary>
</Application.Resources>
</Application>
MainWindow.xaml
各Windowには以下を追加します。
<mah:MetroWindow
省略
xmlns:mah="clr-namespace:MahApps.Metro.Controls;assembly=MahApps.Metro"
省略
>
MainWindow.xaml.cs
MainWindow.xaml.csは以下に変更します。
public partial class MainWindow : MetroWindow
{
// 省略
}
結果
結果は以下の通りです。
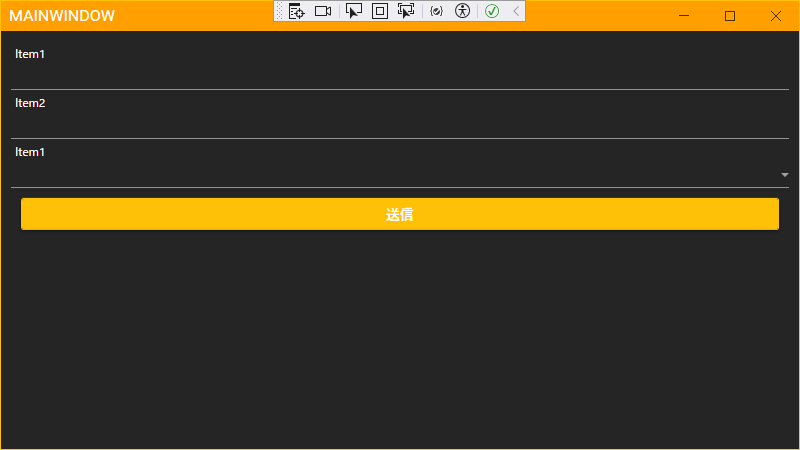
デザイナの背景色が白のまま
こんな感じになっているのでイメージが分かりづらい。
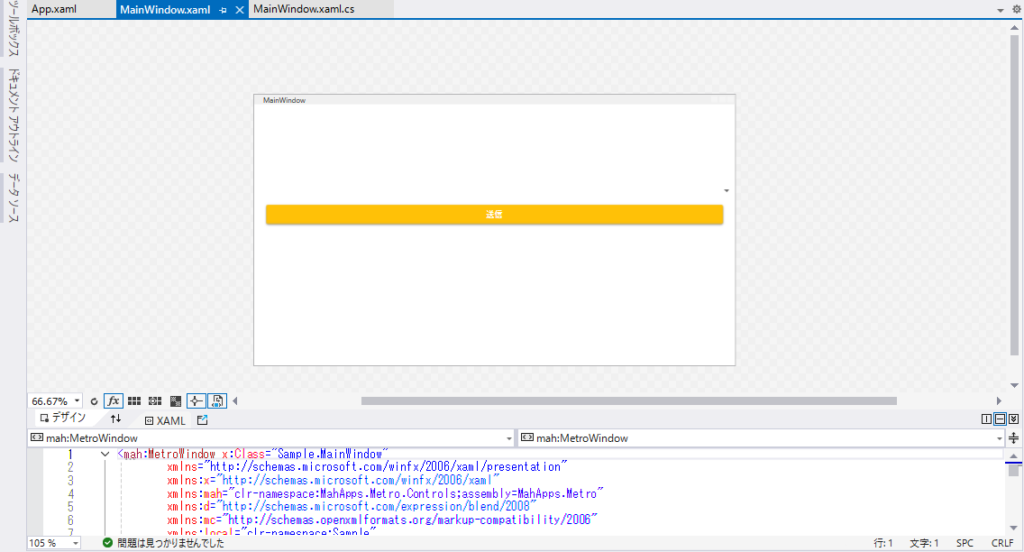
そんな時は.xamlに以下を設定します。
Background="{DynamicResource MahApps.Brushes.Control.Background}"
こんな感じになります。
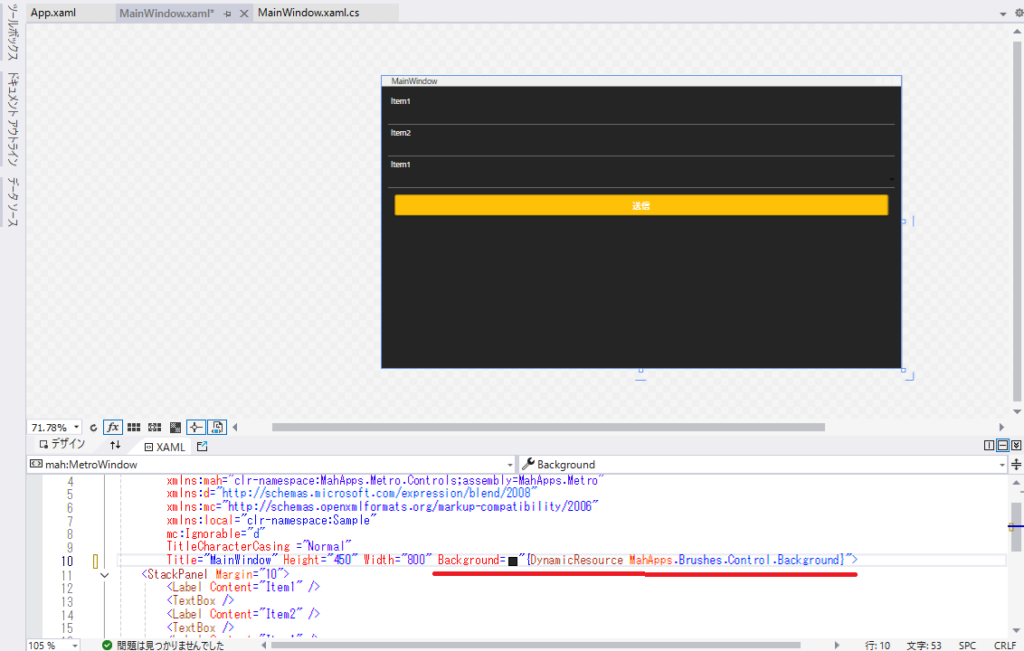
タイトルバーの大文字固定を解除したい
タイトルバーがデフォルトだと大文字固定になります。
MetroWindowはプロパティにTitleCharacterCasingを持っているので以下のように指定します。
<mah:MetroWindow x:Class="Sample.MainWindow"
(省略)
TitleCharacterCasing ="Normal"
(省略)
Title="MainWindow" Height="450" Width="800">
テーマカラーを変更したい
テーマカラーを変更したい場合はApp.xamlの以下を変更します。
<Application x:Class="Sample.App"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:Sample"
StartupUri="MainWindow.xaml">
<Application.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<ResourceDictionary Source="pack://application:,,,/MahApps.Metro;component/Styles/Controls.xaml" />
<!-- Material Design -->
<!-- ↓↓↓ここを修正する↓↓↓ -->
<ResourceDictionary Source="pack://application:,,,/MaterialDesignColors;component/Themes/Recommended/Primary/MaterialDesignColor.Amber.xaml"/>
<ResourceDictionary Source="pack://application:,,,/MaterialDesignColors;component/Themes/Recommended/Secondary/MaterialDesignColor.Cyan.xaml" />
<!-- ↑↑↑ここを修正する↑↑↑ -->
参考
GitHub
差し替え候補
Primary
- MaterialDesignColor.Amber.xaml
- MaterialDesignColor.Blue.xaml
- MaterialDesignColor.BlueGrey.xaml
- MaterialDesignColor.Brown.xaml
- MaterialDesignColor.Cyan.xaml
- MaterialDesignColor.DeepOrange.xaml
- MaterialDesignColor.DeepPurple.xaml
- MaterialDesignColor.Green.xaml
- MaterialDesignColor.Grey.xaml
- MaterialDesignColor.Indigo.xaml
- MaterialDesignColor.LightBlue.xaml
- MaterialDesignColor.LightGreen.xaml
- MaterialDesignColor.Lime.xaml
- MaterialDesignColor.Orange.xaml
- MaterialDesignColor.Pink.xaml
- MaterialDesignColor.Purple.xaml
- MaterialDesignColor.Red.xaml
- MaterialDesignColor.Teal.xaml
- MaterialDesignColor.Yellow.xaml
Secondary
- MaterialDesignColor.Amber.xaml
- MaterialDesignColor.Blue.xaml
- MaterialDesignColor.Cyan.xaml
- MaterialDesignColor.DeepOrange.xaml
- MaterialDesignColor.DeepPurple.xaml
- MaterialDesignColor.Green.xaml
- MaterialDesignColor.Indigo.xaml
- MaterialDesignColor.LightBlue.xaml
- MaterialDesignColor.LightGreen.xaml
- MaterialDesignColor.Lime.xaml
- MaterialDesignColor.Orange.xaml
- MaterialDesignColor.Pink.xaml
- MaterialDesignColor.Purple.xaml
- MaterialDesignColor.Red.xaml
- MaterialDesignColor.Teal.xaml
- MaterialDesignColor.Yellow.xaml
差し替えイメージ
Primaryだけ差し替えたものを参考までに列挙しておきます。
MaterialDesignColor.Amber.xaml
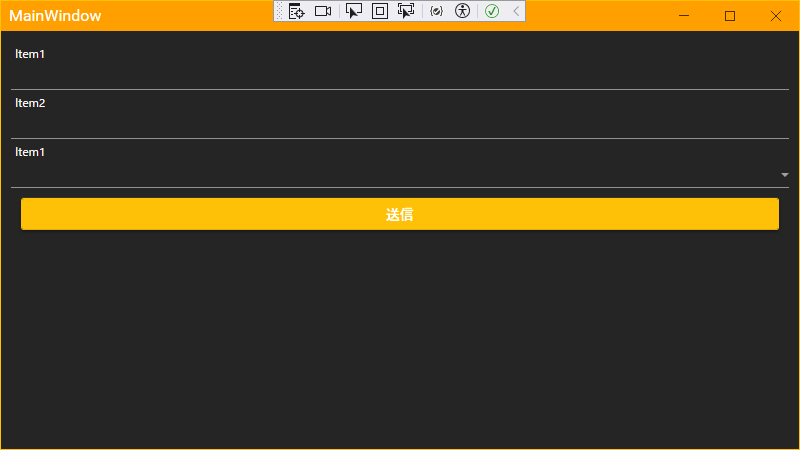
MaterialDesignColor.Blue.xaml
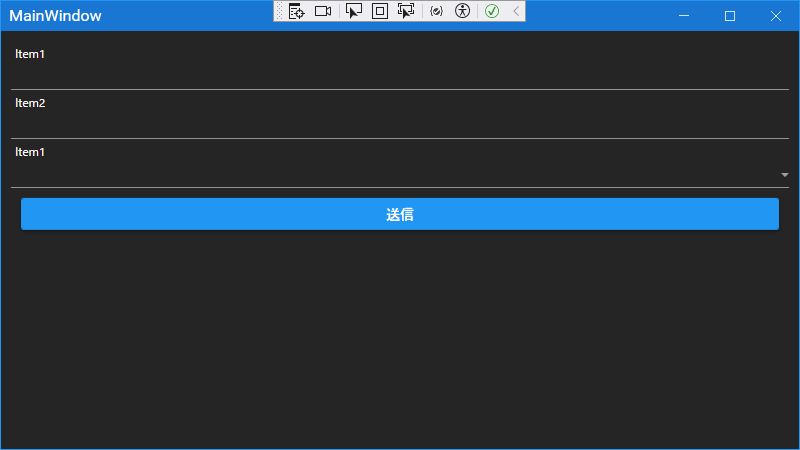
MaterialDesignColor.BlueGrey.xaml
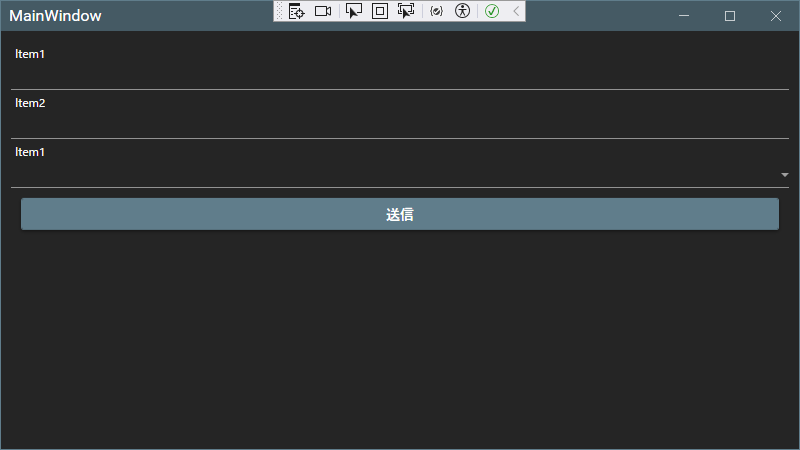
MaterialDesignColor.Brown.xaml
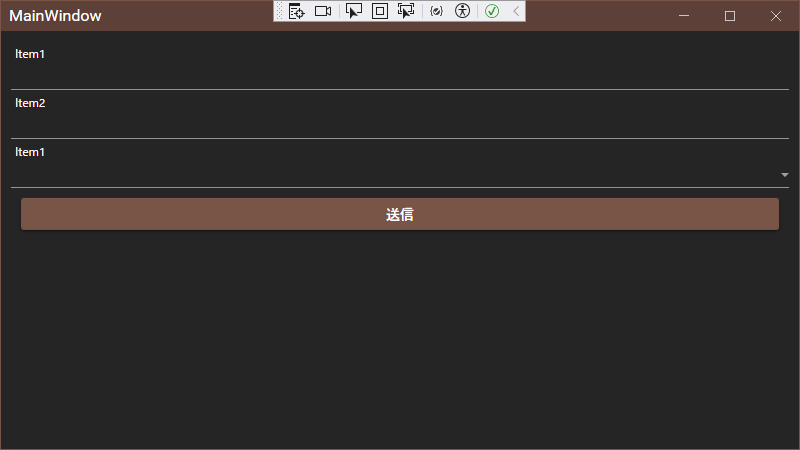
MaterialDesignColor.Cyan.xaml
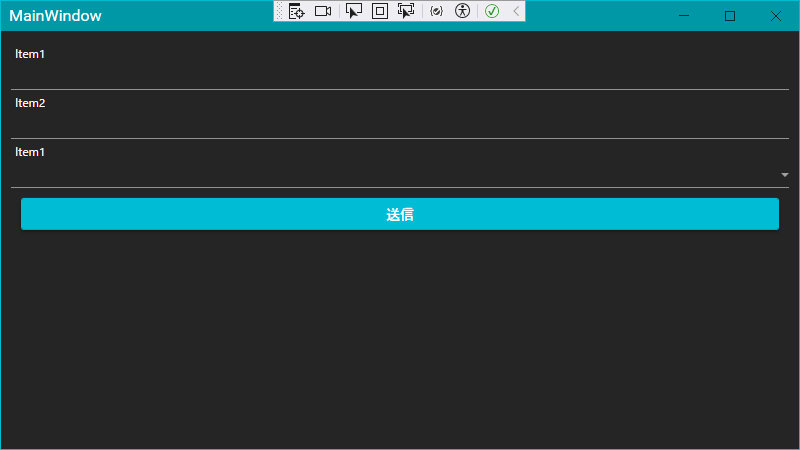
MaterialDesignColor.DeepOrange.xaml
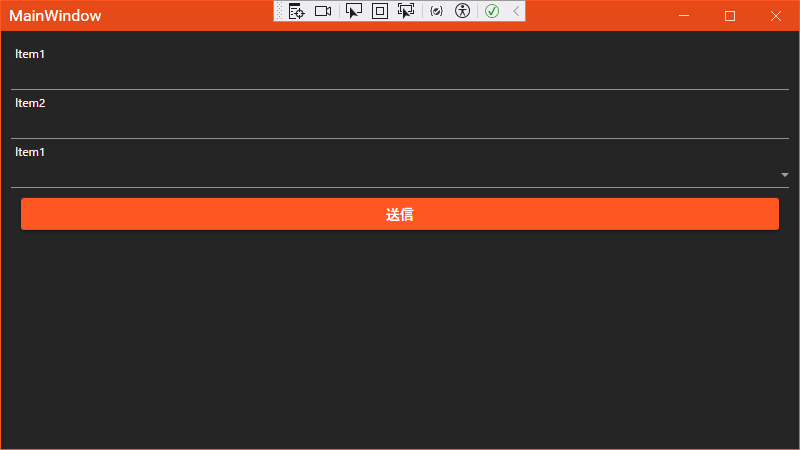
MaterialDesignColor.DeepPurple.xaml
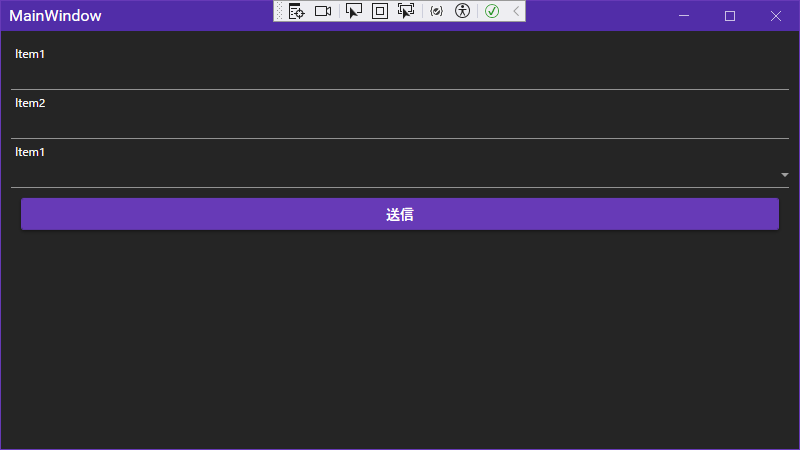
MaterialDesignColor.Green.xaml
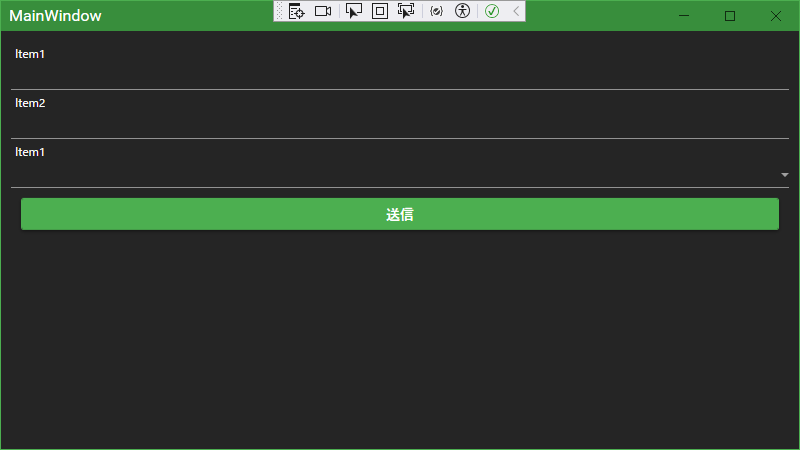
MaterialDesignColor.Grey.xaml
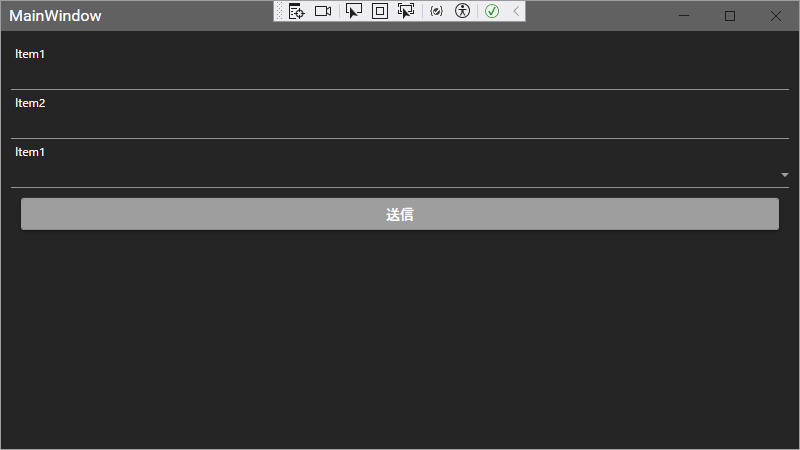
MaterialDesignColor.Indigo.xaml
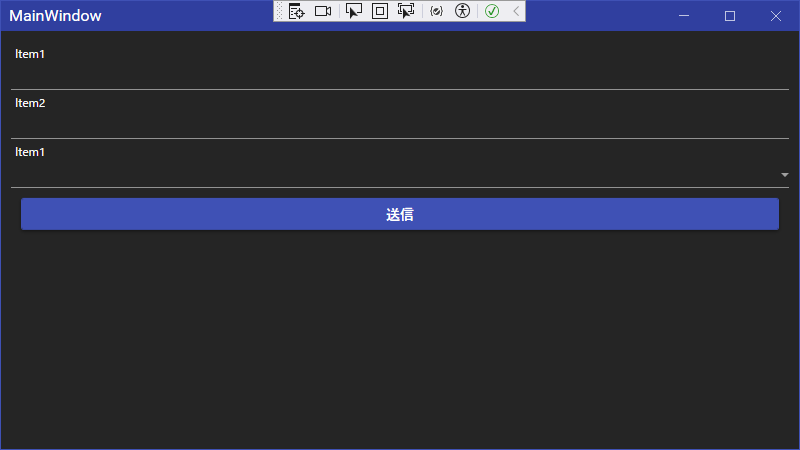
MaterialDesignColor.LightBlue.xaml
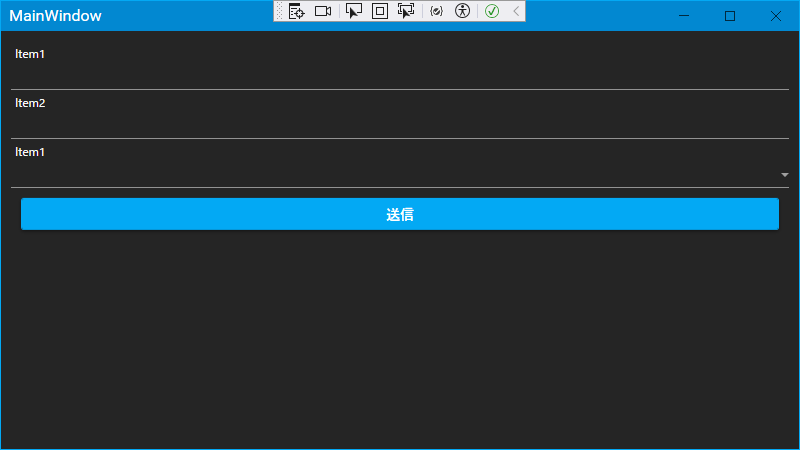
MaterialDesignColor.LightGreen.xaml
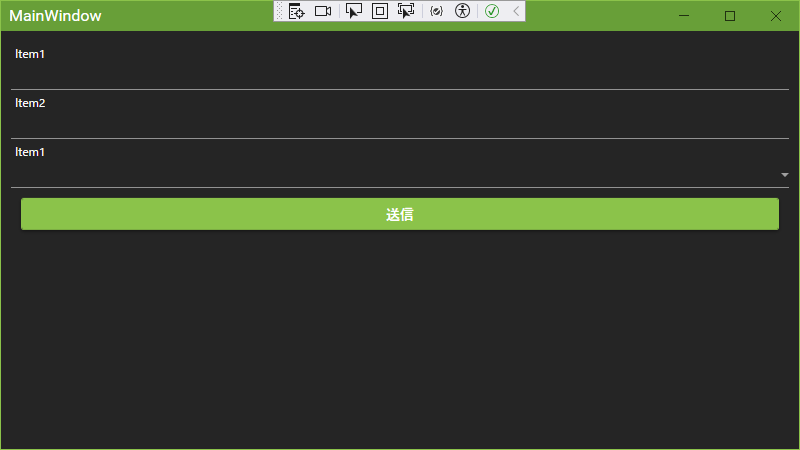
MaterialDesignColor.Lime.xaml
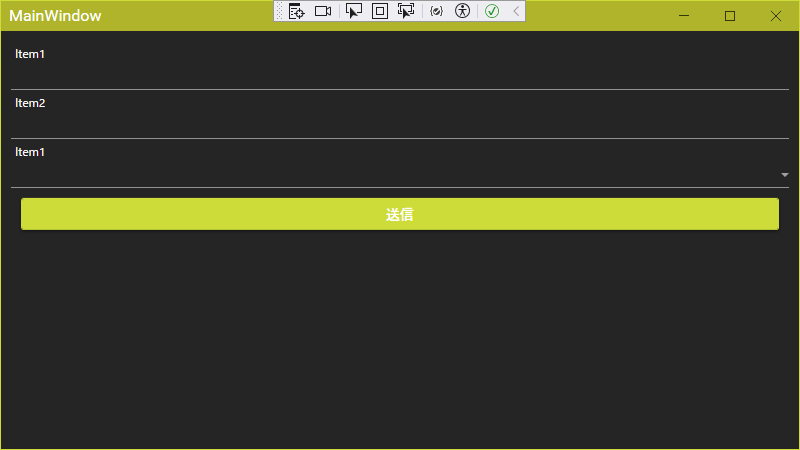
MaterialDesignColor.Orange.xaml
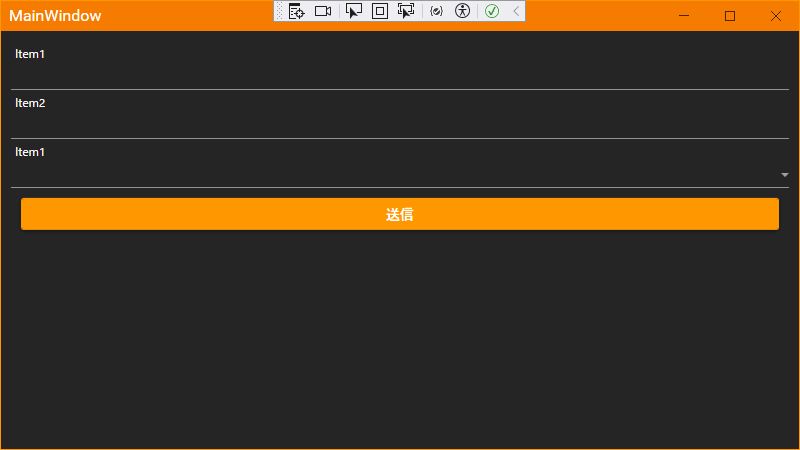
MaterialDesignColor.Pink.xaml
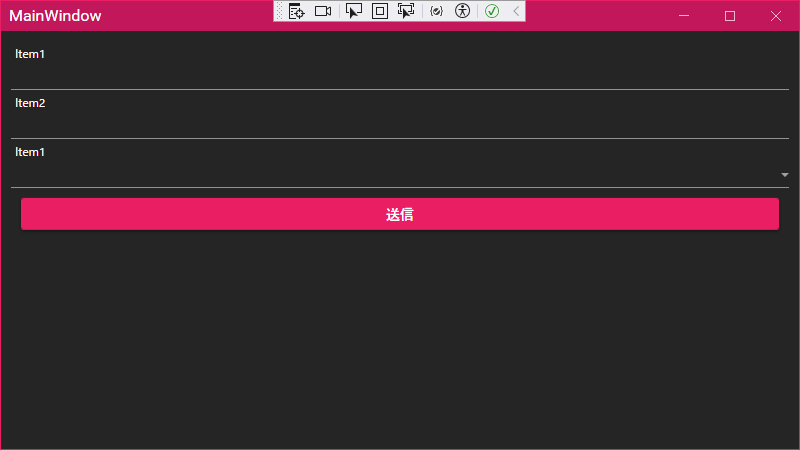
MaterialDesignColor.Purple.xaml
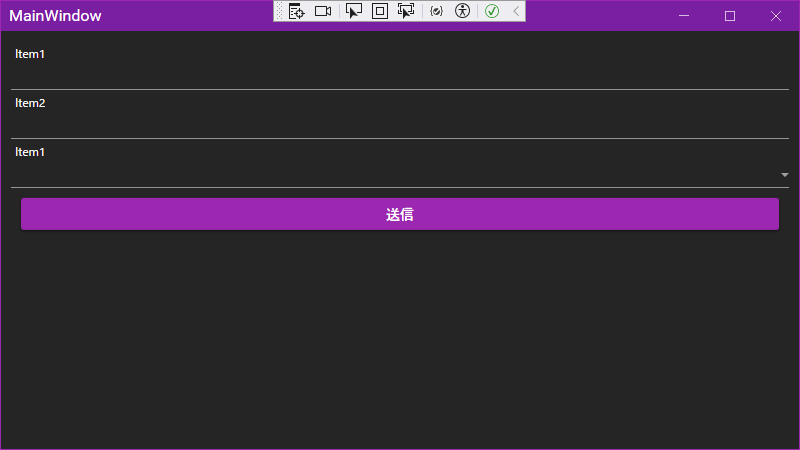
MaterialDesignColor.Red.xaml
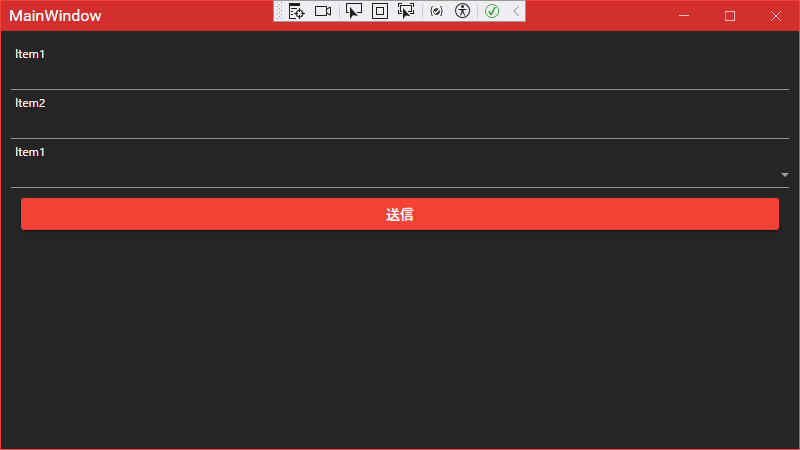
MaterialDesignColor.Teal.xaml
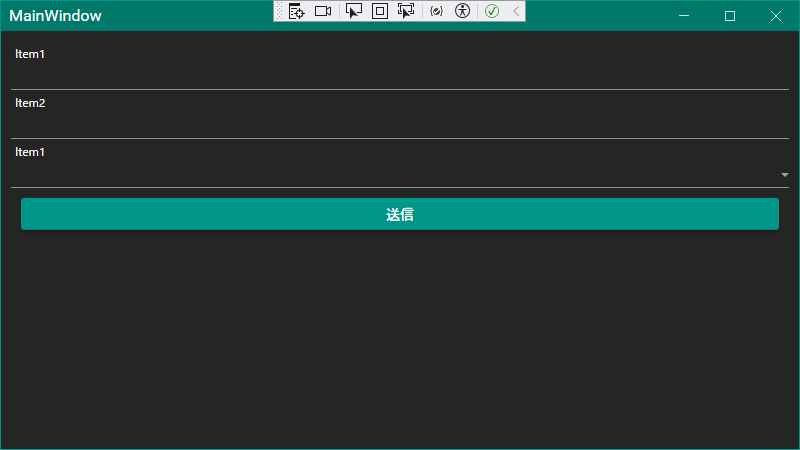
MaterialDesignColor.Yellow.xaml
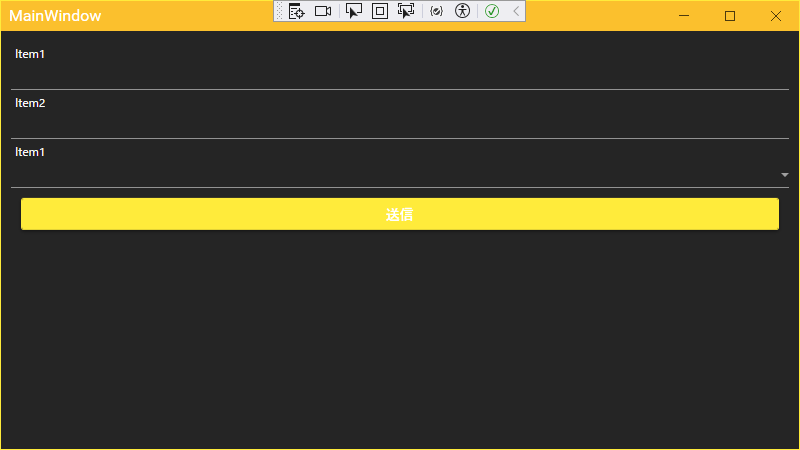
以上
コメントを残す